Webbrowser Tutorial
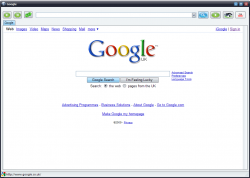
Ok to make this you will need to start a new project and add the following to your form with these properties.....
Imports System.Net
Public Class Form1
Dim i As Integer = 0
Public tabs = 0
Public thisurl
'This button loads a webpage inside the current selected tab
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
CType(TabControl1.SelectedTab.Controls.Item(0), WebBrowser).Navigate(ComboBox1.Text)
Try
Dim iconURL = "http://" & ComboBox1.Text & "/favicon.ico"
Dim request As System.Net.WebRequest = System.Net.HttpWebRequest.Create(iconURL)
Dim response As System.Net.HttpWebResponse = request.GetResponse()
Dim stream As System.IO.Stream = response.GetResponseStream()
Dim favicon = Image.FromStream(stream)
ToolStripStatusLabel1.Image = (favicon)
Catch ex As Exception
ToolStripStatusLabel1.Image = My.Resources.Globe
End Try
If ComboBox1.Text <> ""And ComboBox1.FindStringExact(ComboBox1.Text) < 0 Then
ComboBox1.Items.Add(ComboBox1.Text)
With ComboBox1
Dim i As Integer
Dim s As String = ""
For i = 0 To .Items.Count - 1
s += .Items(i).ToString & vbNewLine
Next
My.Computer.FileSystem.WriteAllText(Application.StartupPath & "\visited.urls", s, False)
End With
End If
End Sub
'This button creates a new tab with new webpage inside of it
Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
Try
Dim iconURL = "http://" & ComboBox1.Text & "/favicon.ico"
Dim request As System.Net.WebRequest = System.Net.HttpWebRequest.Create(iconURL)
Dim response As System.Net.HttpWebResponse = request.GetResponse()
Dim stream As System.IO.Stream = response.GetResponseStream()
Dim favicon = Image.FromStream(stream)
ToolStripStatusLabel1.Image = (favicon)
Catch ex As Exception
ToolStripStatusLabel1.Image = My.Resources.Globe
End Try
Dim t As New TabPage
Dim w As New WebBrowser
If ComboBox1.Text <> ""And ComboBox1.FindStringExact(ComboBox1.Text) < 0 Then
ComboBox1.Items.Add(ComboBox1.Text)
With ComboBox1
Dim i As Integer
Dim s As String = ""
For i = 0 To .Items.Count - 1
s += .Items(i).ToString & vbNewLine
Next
My.Computer.FileSystem.WriteAllText(Application.StartupPath & "\visited.urls", s, False)
End With
End If
t.Text = ("")
w.Dock = DockStyle.Fill
w.Navigate(ComboBox1.Text)
t.Controls.Add(w)
AddHandler w.DocumentCompleted, AddressOf SetTitle
Me.TabControl1.Controls.Add(t)
TabControl1.SelectTab(t)
tabs += 1
End Sub
'This button goes to homepage
Private Sub Button3_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button3.Click
CType(TabControl1.SelectedTab.Controls.Item(0), WebBrowser).Navigate("www.google.com")
End Sub
'This button downloads the youtube videos
Private Sub Button4_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button4.Click
CType(TabControl1.SelectedTab.Controls.Item(0), WebBrowser).Navigate("javascript:if(document.location.href.match(/http:\/\/[a-zA-Z\.]*youtube\.com\/watch/)){document.location.href='http://www.youtube.com/get_video?fmt='+(isHDAvailable?'22':'18')+'&video_id='+swfArgs['video_id']+'&t='+swfArgs['t']}")
End Sub
'This button goes back 1 page
Private Sub Button5_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button5.Click
CType(TabControl1.SelectedTab.Controls.Item(0), WebBrowser).GoBack()
End Sub
'This button goes forward 1 page
Private Sub Button6_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button6.Click
CType(TabControl1.SelectedTab.Controls.Item(0), WebBrowser).GoForward()
End Sub
'This button refreshes the page
Private Sub Button7_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button7.Click
CType(TabControl1.SelectedTab.Controls.Item(0), WebBrowser).Refresh()
End Sub
'This happens when the program is first started and opens the homepage & loads all saved URL's
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) HandlesMyBase.Load
IfMy.Computer.FileSystem.FileExists(Application.StartupPath & "\visited.urls") Then
Using sr As New IO.StreamReader(Application.StartupPath & "\visited.urls")
Dim item As String = sr.ReadLine
While item <> Nothing
ComboBox1.Items.Add(item)
item = sr.ReadLine
End While
End Using
End If
ComboBox1.AutoCompleteMode = AutoCompleteMode.SuggestAppend
ComboBox1.AutoCompleteSource = AutoCompleteSource.ListItems
ComboBox1.Focus()
Dim t As New TabPage
Dim w As New WebBrowser
t.Text = ("")
w.Dock = DockStyle.Fill
w.Navigate("www.google.com")
t.Controls.Add(w)
AddHandler w.DocumentCompleted, AddressOf SetTitle
Me.TabControl1.Controls.Add(t)
TabControl1.SelectTab(t)
ToolStripStatusLabel1.Text = thisurl
tabs += 1
End Sub
'This shows the webpage name once it is loaded - like: Yahoo! UK & Ireland
Public Sub SetTitle(ByVal sender As Object, ByVal e As Windows.Forms.WebBrowserDocumentCompletedEventArgs)
TabControl1.SelectedTab.Text = CType(TabControl1.SelectedTab.Controls.Item(0), webBrowser).DocumentTitle
Me.Text = CType(TabControl1.SelectedTab.Controls.Item(0), WebBrowser).DocumentTitle
thisurl = CType(TabControl1.SelectedTab.Controls.Item(0), WebBrowser).Url.ToString
ToolStripStatusLabel1.Text = thisurl
Try
Dim iconURL = thisurl & "/favicon.ico"
Dim request As System.Net.WebRequest = System.Net.HttpWebRequest.Create(iconURL)
Dim response As System.Net.HttpWebResponse = request.GetResponse()
Dim stream As System.IO.Stream = response.GetResponseStream()
Dim favicon = Image.FromStream(stream)
ToolStripStatusLabel1.Image = (favicon)
Catch ex As Exception
ToolStripStatusLabel1.Image = My.Resources.Globe
End Try
If InStr(thisurl, "/watch?v=") > 0 Then
Button4.Enabled = True
Else
Button4.Enabled = False
End If
End Sub
'This closes the tab if you click it twice
Private Sub TabControl1_MouseDoubleClick(ByVal sender As System.Object, ByVal e As System.Windows.Forms.MouseEventArgs) Handles TabControl1.MouseDoubleClick
If tabs > 1 Then
TabControl1.TabPages.RemoveAt(TabControl1.SelectedIndex)
tabs -= 1
End If
End Sub
'This updates webpage title when moving from tab to tab
Private Sub TabControl1_MouseClick(ByVal sender As System.Object, ByVal e As System.Windows.Forms.MouseEventArgs) Handles TabControl1.MouseClick
Me.Text = CType(TabControl1.SelectedTab.Controls.Item(0), WebBrowser).DocumentTitle
thisurl = CType(TabControl1.SelectedTab.Controls.Item(0), WebBrowser).Url.ToString
If InStr(thisurl, "/watch?v=") > 0 Then
Button4.Enabled = True
Else
Button4.Enabled = False
End If
End Sub
End Class
- Button1(main search button)
- Button2(add new tab button)
- Button3(goto home page)
- Button4(Youtube download) - Make button enabled to false
- Button5(go back a page)
- Button6(go forward a page)
- Button7(refresh a page)
- Combobox(for the address bar)
- Tab Control(for the webpages) - Remove both tabs
- StatusStrip - add a toolstripstatuslabel to it by right-mouse click
Imports System.Net
Public Class Form1
Dim i As Integer = 0
Public tabs = 0
Public thisurl
'This button loads a webpage inside the current selected tab
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
CType(TabControl1.SelectedTab.Controls.Item(0), WebBrowser).Navigate(ComboBox1.Text)
Try
Dim iconURL = "http://" & ComboBox1.Text & "/favicon.ico"
Dim request As System.Net.WebRequest = System.Net.HttpWebRequest.Create(iconURL)
Dim response As System.Net.HttpWebResponse = request.GetResponse()
Dim stream As System.IO.Stream = response.GetResponseStream()
Dim favicon = Image.FromStream(stream)
ToolStripStatusLabel1.Image = (favicon)
Catch ex As Exception
ToolStripStatusLabel1.Image = My.Resources.Globe
End Try
If ComboBox1.Text <> ""And ComboBox1.FindStringExact(ComboBox1.Text) < 0 Then
ComboBox1.Items.Add(ComboBox1.Text)
With ComboBox1
Dim i As Integer
Dim s As String = ""
For i = 0 To .Items.Count - 1
s += .Items(i).ToString & vbNewLine
Next
My.Computer.FileSystem.WriteAllText(Application.StartupPath & "\visited.urls", s, False)
End With
End If
End Sub
'This button creates a new tab with new webpage inside of it
Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
Try
Dim iconURL = "http://" & ComboBox1.Text & "/favicon.ico"
Dim request As System.Net.WebRequest = System.Net.HttpWebRequest.Create(iconURL)
Dim response As System.Net.HttpWebResponse = request.GetResponse()
Dim stream As System.IO.Stream = response.GetResponseStream()
Dim favicon = Image.FromStream(stream)
ToolStripStatusLabel1.Image = (favicon)
Catch ex As Exception
ToolStripStatusLabel1.Image = My.Resources.Globe
End Try
Dim t As New TabPage
Dim w As New WebBrowser
If ComboBox1.Text <> ""And ComboBox1.FindStringExact(ComboBox1.Text) < 0 Then
ComboBox1.Items.Add(ComboBox1.Text)
With ComboBox1
Dim i As Integer
Dim s As String = ""
For i = 0 To .Items.Count - 1
s += .Items(i).ToString & vbNewLine
Next
My.Computer.FileSystem.WriteAllText(Application.StartupPath & "\visited.urls", s, False)
End With
End If
t.Text = ("")
w.Dock = DockStyle.Fill
w.Navigate(ComboBox1.Text)
t.Controls.Add(w)
AddHandler w.DocumentCompleted, AddressOf SetTitle
Me.TabControl1.Controls.Add(t)
TabControl1.SelectTab(t)
tabs += 1
End Sub
'This button goes to homepage
Private Sub Button3_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button3.Click
CType(TabControl1.SelectedTab.Controls.Item(0), WebBrowser).Navigate("www.google.com")
End Sub
'This button downloads the youtube videos
Private Sub Button4_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button4.Click
CType(TabControl1.SelectedTab.Controls.Item(0), WebBrowser).Navigate("javascript:if(document.location.href.match(/http:\/\/[a-zA-Z\.]*youtube\.com\/watch/)){document.location.href='http://www.youtube.com/get_video?fmt='+(isHDAvailable?'22':'18')+'&video_id='+swfArgs['video_id']+'&t='+swfArgs['t']}")
End Sub
'This button goes back 1 page
Private Sub Button5_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button5.Click
CType(TabControl1.SelectedTab.Controls.Item(0), WebBrowser).GoBack()
End Sub
'This button goes forward 1 page
Private Sub Button6_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button6.Click
CType(TabControl1.SelectedTab.Controls.Item(0), WebBrowser).GoForward()
End Sub
'This button refreshes the page
Private Sub Button7_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button7.Click
CType(TabControl1.SelectedTab.Controls.Item(0), WebBrowser).Refresh()
End Sub
'This happens when the program is first started and opens the homepage & loads all saved URL's
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) HandlesMyBase.Load
IfMy.Computer.FileSystem.FileExists(Application.StartupPath & "\visited.urls") Then
Using sr As New IO.StreamReader(Application.StartupPath & "\visited.urls")
Dim item As String = sr.ReadLine
While item <> Nothing
ComboBox1.Items.Add(item)
item = sr.ReadLine
End While
End Using
End If
ComboBox1.AutoCompleteMode = AutoCompleteMode.SuggestAppend
ComboBox1.AutoCompleteSource = AutoCompleteSource.ListItems
ComboBox1.Focus()
Dim t As New TabPage
Dim w As New WebBrowser
t.Text = ("")
w.Dock = DockStyle.Fill
w.Navigate("www.google.com")
t.Controls.Add(w)
AddHandler w.DocumentCompleted, AddressOf SetTitle
Me.TabControl1.Controls.Add(t)
TabControl1.SelectTab(t)
ToolStripStatusLabel1.Text = thisurl
tabs += 1
End Sub
'This shows the webpage name once it is loaded - like: Yahoo! UK & Ireland
Public Sub SetTitle(ByVal sender As Object, ByVal e As Windows.Forms.WebBrowserDocumentCompletedEventArgs)
TabControl1.SelectedTab.Text = CType(TabControl1.SelectedTab.Controls.Item(0), webBrowser).DocumentTitle
Me.Text = CType(TabControl1.SelectedTab.Controls.Item(0), WebBrowser).DocumentTitle
thisurl = CType(TabControl1.SelectedTab.Controls.Item(0), WebBrowser).Url.ToString
ToolStripStatusLabel1.Text = thisurl
Try
Dim iconURL = thisurl & "/favicon.ico"
Dim request As System.Net.WebRequest = System.Net.HttpWebRequest.Create(iconURL)
Dim response As System.Net.HttpWebResponse = request.GetResponse()
Dim stream As System.IO.Stream = response.GetResponseStream()
Dim favicon = Image.FromStream(stream)
ToolStripStatusLabel1.Image = (favicon)
Catch ex As Exception
ToolStripStatusLabel1.Image = My.Resources.Globe
End Try
If InStr(thisurl, "/watch?v=") > 0 Then
Button4.Enabled = True
Else
Button4.Enabled = False
End If
End Sub
'This closes the tab if you click it twice
Private Sub TabControl1_MouseDoubleClick(ByVal sender As System.Object, ByVal e As System.Windows.Forms.MouseEventArgs) Handles TabControl1.MouseDoubleClick
If tabs > 1 Then
TabControl1.TabPages.RemoveAt(TabControl1.SelectedIndex)
tabs -= 1
End If
End Sub
'This updates webpage title when moving from tab to tab
Private Sub TabControl1_MouseClick(ByVal sender As System.Object, ByVal e As System.Windows.Forms.MouseEventArgs) Handles TabControl1.MouseClick
Me.Text = CType(TabControl1.SelectedTab.Controls.Item(0), WebBrowser).DocumentTitle
thisurl = CType(TabControl1.SelectedTab.Controls.Item(0), WebBrowser).Url.ToString
If InStr(thisurl, "/watch?v=") > 0 Then
Button4.Enabled = True
Else
Button4.Enabled = False
End If
End Sub
End Class